LOG – Write a log message
This block writes a user-defined message in the log module of Cockpit.
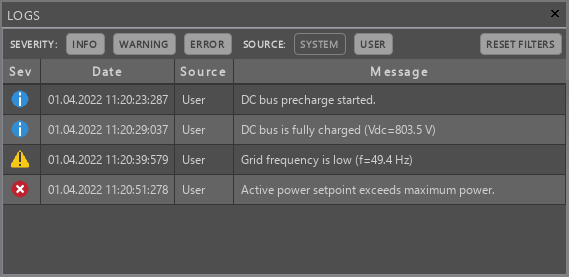
Numerical values can be inserted into the message using the conversion specifier “%f
“, that will take to the value of the second input signal. This block only supports floating-point numbers. Optionally, the number of digits displayed after the decimal point can be specified using the precision modifier "."
. Here are some examples:
Log message field | Input value | Message displayed in the log module |
---|---|---|
The value is: %f | 3.141592653 | The value is: 3.141593 |
The value is: %.3f | 3.141592653 | The value is: 3.142 |
The value is: %.0f | 3.141592653 | The value is: 3 |
The value is: %f | 3 | The value is: 3.000000 |
The value is: %.3f | 3 | The value is: 3.000 |
The value is: %.0f | 3 | The value is: 3 |
Simulink block
Signal specification
- The first input is a trigger port that generates a log message when its value rises from a negative or zero value to a positive value.
- The second input is only visible when
Include variables in message
is checked. It is used to input numerical value(s) into the message. The value(s) are sampled when the log message is triggered.
Its width is defined by the parameterNumber of variables
and must also correspond to the number of conversion specifiers contained in the message.
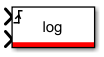
Parameters
Log message
is the message that will be displayed in the log module of Cockpit.String type
indicates whether theLog message
field contains a literal text string or an expression that should be evaluated.Severity
defines the type of message (Info, Warning, or Error). The log module of Cockpit lets you filter the displayed message by severity.Include variables in message
indicates if numerical values are inserted in the message using the%f
placeholder. If checked, a second port appears to input the numerical values.Number of variables
is the number of numerical values that are inserted into the message. This field is only visible ifInclude variables in message
is checked.
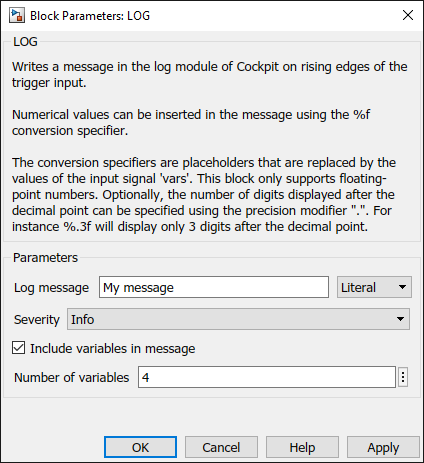
PLECS block
Signal specification
- The first input is a trigger port that generates a log message when its value rises from a negative or zero value to a positive value.
- The second input is only visible when
Include variables in message
is checked. It is used to input numerical value(s) into the message. The value(s) are sampled when the log message is triggered. - Its width is defined by the parameter
Number of variables
and must also correspond to the number of conversion specifiers contained in the message.
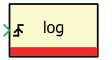
Parameters
Log message
is the message that will be displayed in the log module of Cockpit.String type
indicates whether theLog message
field contains a literal text string or an expression that should be evaluated.Severity
defines the type of message (Info, Warning, or Error). The log module of Cockpit lets you filter the displayed message by severity.Include variables in message
indicates if numerical values are inserted in the message using the%f
placeholder. If checked, a second port appears to input the numerical values.Number of variables
is the number of numerical values that are inserted into the message. This field is only visible ifInclude variables in message
is checked.
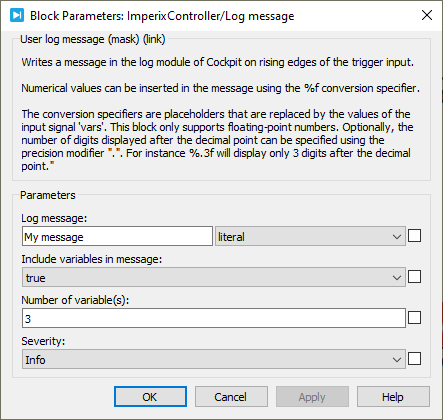
C++ functions
Example of use
tUserSafe UserInit(void)
{
//...
// Configure a warning message with a unique id of 0
Log_AddMsg(0, 20, "Operating limits exceeded (V=%.3fV / I=%.3fA)");
//...
return SAFE;
}
tUserSafe UserInterrupt(void)
{
//...
static bool warning_message_sent = false;
if(V_meas > 850 || I_meas > 40){
float log_values[2];
log_values[0] = V_meas;
log_values[1] = I_meas;
// Display the message in Cockpit
if(!warning_message_sent) {
Log_SendMsg(0, log_values, 2);
warning_message_sent = true;
}
} else {
warning_message_sent= false;
}
//...
return SAFE;
}
Code language: C++ (cpp)