PI controller implementation for current control
Table of Contents
This technical note presents a possible current control implementation for power converters.
First, the note introduces the general operating principles of a simple Proportional-Integral PI controller. Then, an example of current control for a boost converter is provided. Finally, a practical control implementation is introduced, targeting the B-Box RCP or B-Board PRO with both C/C++ and automated code generation approaches.
Software resources
General principles of PI control
PI controllers are widely used in power electronics, thanks to their simple structure and implementation. They are very frequently used to control constant or slowly-varying quantities, e.g. in direct current (DC) applications, as well as in AC applications together with coordinate transformations. When implemented in a parallel form, a PI controller can be represented as shown below:
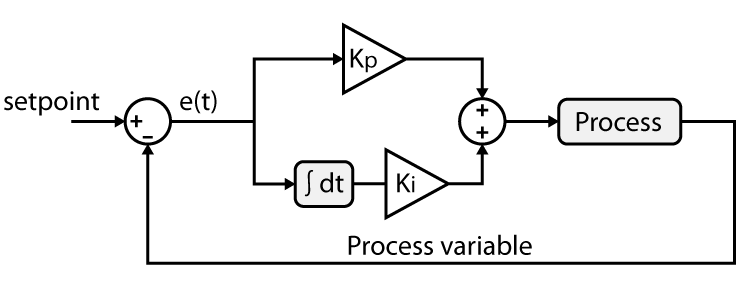
PI controller for DC current regulation example
The simplest and most obvious example of PI-based control is certainly given by the control of the inductor current within a boost-type converter.
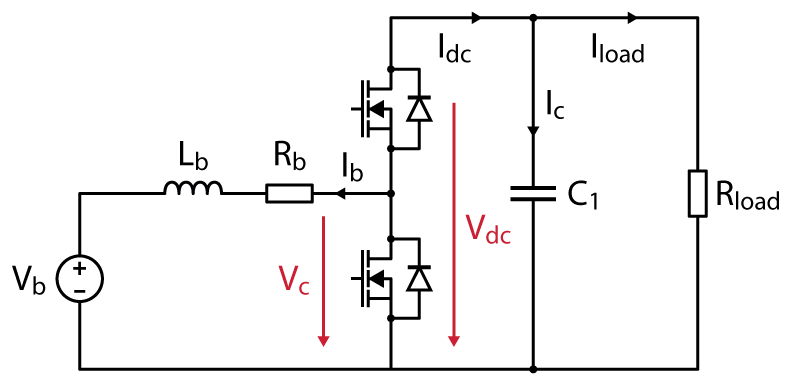
In this system, basic circuit equations show that the inductor current is given by:
$$R_b I_b + L_b \displaystyle\frac{d I_b}{dt} = V_L = V_b – V_c$$
In the Laplace domain, this translates into:
$$I_b = \displaystyle\frac{1}{R_b + s L_b} \cdot V_L$$
System-level modeling
Plant
A widely-accepted model for the proposed system is shown below. Four distinct parts can be clearly identified.
$$P_1(s) = \displaystyle\frac{K_1}{1+s T_1} \qquad \text{with} \qquad \begin{cases}K_1 = 1/R_b \\ T_1 = L_b/R_b \end{cases}$$
Measurements
The measurements of the current and the voltages \(I_b\), \(V_b\) and \(V_{dc}\) are generally modeled using a low-pass filter approximation, or they are neglected. The sampling corresponds to a zero-order hold (ZOH) which introduces a lag, which is the sampling delay.
Control
The control algorithm consists of a digital PI controller followed by some basic mathematic operations to compute the duty cycle. The whole algorithm requires a certain amount of computation time, which is represented as a delay.
Modulation
The Pulse-Width Modulation (PWM) is also generally modeled as a simple delay.
Digital implementation if a PI controller
The three most common implementations [2] of the PI controller are the following:
$$ \begin{aligned}
C(z) &= K_p + K_i T_s \frac{1}{z-1} & \text{Forward Euler} \\
&= K_p + K_i T_s \frac{z}{z-1} & \text{Backward Euler} \\
&= K_p + K_i \frac{T_s}{2} \frac{z+1}{z-1} & \text{Tustin /Trapezoidal} \\
\end{aligned}$$
The parameter \(T_s\) corresponds to the period of the interruption. The advantages/disadvantages of these different discretizations are well discussed in [1]. The Forward Euler will be used in this note, and the corresponding difference equation is given below.
$$\begin{aligned} y(k) &= P(k) + I(k) \\ P(k) &= K_p \cdot e(k) \\ I(k) &= I(k-1) + K_i \cdot T_s \cdot e(k-1) \end{aligned}$$
These equations can be easily used for generating run-time code.
Tuning and performance evaluation
Different methods are used in the literature to determine the parameters of a PI controller. Those methods are well detailed and explained in [1]. In this note, the Magnitude Optimum (MO) will be used.
The goal of the MO is to make the frequency response from reference to the plant output as close to one as possible for low frequencies. The controller parameters are defined as:
$$\begin{aligned}
&T_n = T_1 \\
&T_i = 2 K_1 T_{d,tot}\\
& \text{and}\\
&K_p = T_n /T_i \\
&K_i = 1 / T_i
\end{aligned}$$
The parameter \(T_{d,tot}\) represents the sum of all the small delays in the system, such as the sampling delay or the modulation delay mentioned above. The product note PN142 explains how to determine the total delay of the system.
Academic references
[1] Karl J. Åström and Tore Hägglund; “Advanced PID Control”; 1995
[2] Dew Toochinda; “Discrete-time PID Controller Implementation”; SciLab.org; 2015
B-Box / B-Board implementation
C/C++ code
The imperix IDE provides numerous pre-written and pre-optimized functions. Controllers such as P, PI, PID and PR are already available and can be found in the controllers.h/.cpp
files.
As for all controllers, PI controllers are based on:
- A pseudo-object
PIDcontroller
, which contains pre-computed parameters as well as state variables. - A configuration function, meant to be called during
UserInit()
, namedConfigPIDController()
. - A run-time function meant to be called during the user-level ISR such as
UserInterrupt()
, namedRunPIController()
.
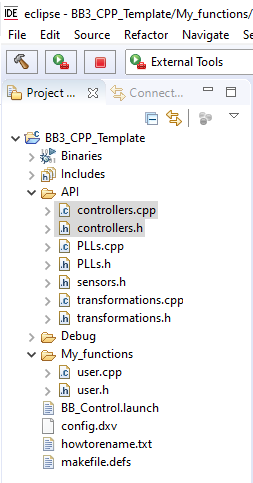
Implementation example of PI controller
#include "../API/controllers.h"
PIDController mycontroller;
float Kp = 13.64;
float Ki = 120.00;
float limup = 500;
float limlow = -500;
tUserSafe UserInit(void)
{
//... some code
ConfigPIDController(&mycontroller, Kp, Ki, 0, limup, limlow, SAMPLING_PERIOD, 0);
//... some code
return SAFE;
}
Code language: C++ (cpp)
tUserSafe UserInterrupt(void)
{
//... some code
UL_ref = RunPIController(&mycontroller, Ib_ref - Ib);
//... some code
return SAFE;
}
Code language: C++ (cpp)
Simulink implementation
The enclosed file provides an implementation example of the whole control loop, including a PI controller. Of course, the standard “Discrete PID Controller” block can also be used.
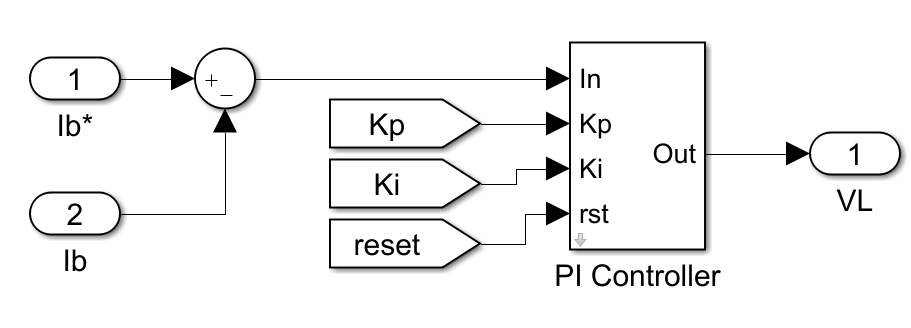
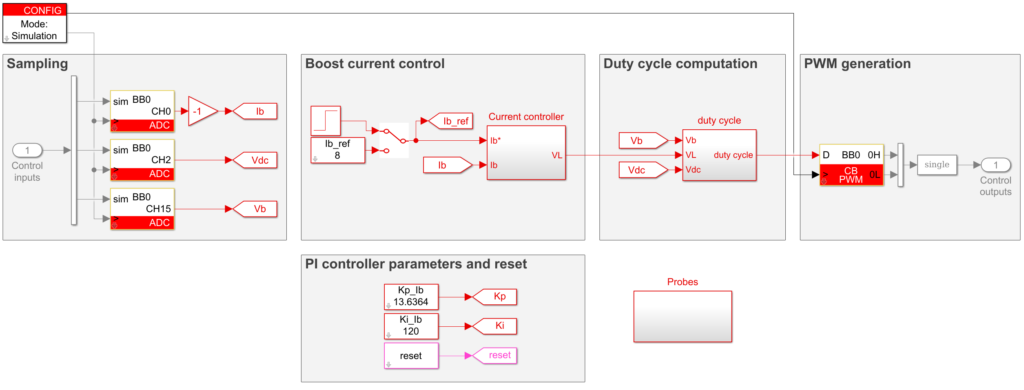
PLECS implementation
The included file for PLECS also provides a PI block. The default PI block of the PLECS library can also be used.
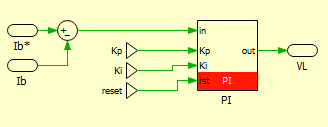
Results
The PI current control was tested in the case of a boost converter connected to a resistive load. A current reference step was performed in both simulation (with Simulink) and experimental modes. The following graph shows a comparison between both results:
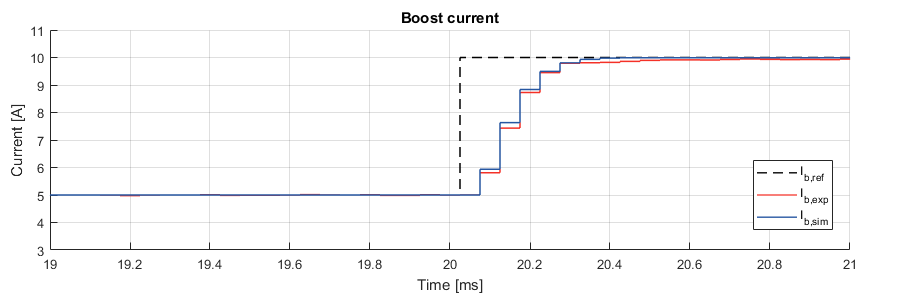